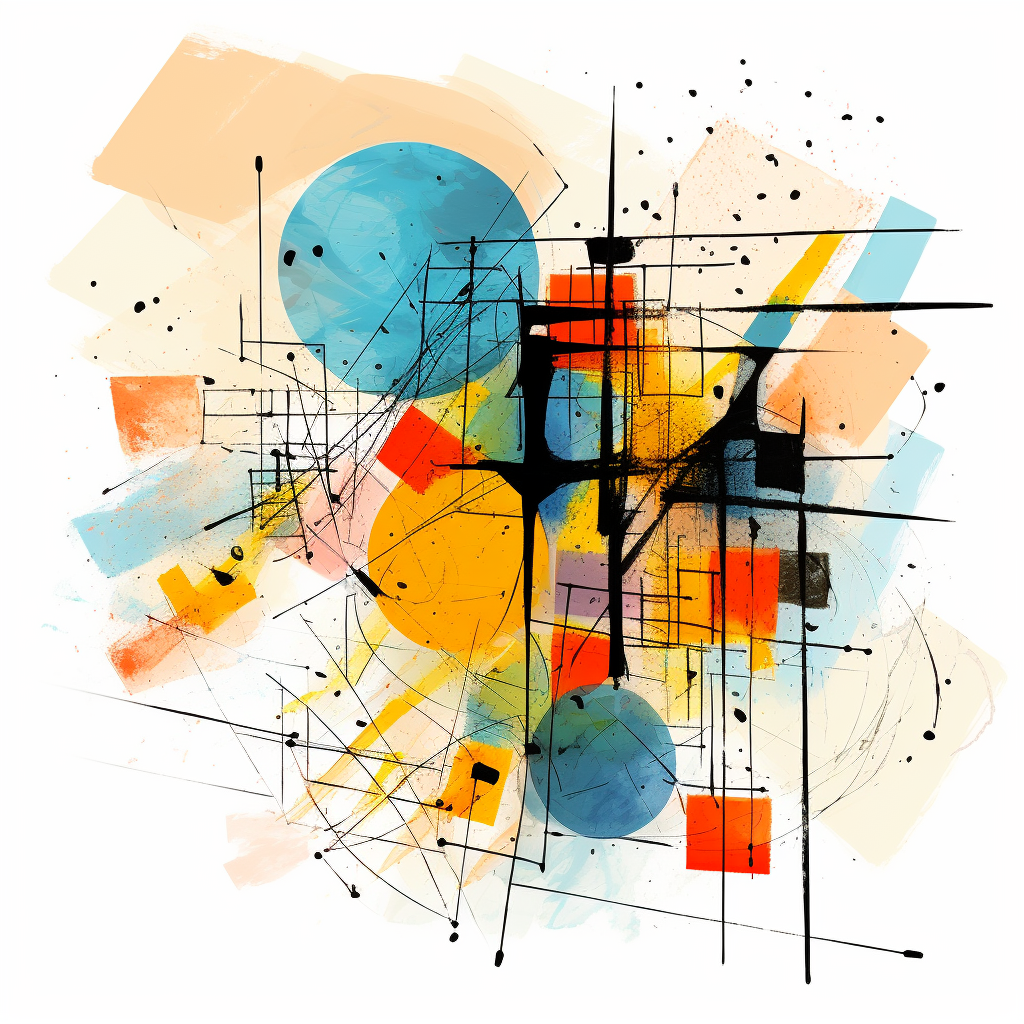
Definition
All told, a monad in X is just a monoid in the category of endofunctors of X, with product × replaced by composition of endofunctors and unit set by the identity endofunctor.
Categories for the Working Mathematician
Or simplified:
A monad is just a monoid in the category of endofunctors
Anonymous
The second phrase has almost reached the status of a software engineering meme, spoken in hushed reverential tones or sometimes with a wink, as if to confound newcomers and solidify the mystique surrounding functional programming. Yet, the statement is not mere jargon or an intellectual gatekeeping mechanism — it’s a precise description that holds significant meaning for those willing to unravel its layers.
Let’s dissect this intimidating phrase, shall we? At its core, it draws upon three major concepts: Monads, Monoids, and Endofunctors. These terms may seem like characters in a mathematical drama, but they serve very functional (pun intended) roles in programming, particularly in languages that favor functional paradigms.
Monoids
A monoid is an algebraic structure with a set of elements, a binary operation, and an identity element. The binary operation must be associative, and when applied to the identity element, it leaves any other element unchanged. In programming, think of simple operations like string concatenation or addition — these are monoids in action.
Endofunctors
An endofunctor is a mapping within a single category. For those not well-versed in category theory, consider it as a higher-level abstraction of a function: it not only transforms elements from one set to another but also respects the relationships between these elements (like function composition). In programming, a functor often manifests as a data structure that supports map-like operations, enabling transformations while maintaining its shape.
Monads
Now we arrive at the pièce de résistance: the Monad. Monads exist as a special kind of endofunctor equipped with two additional operations — unit and bind — that adhere to specific laws. In essence, a monad is a design pattern that allows you to handle program-wide concerns (like state or I/O) in a pure functional way.
By understanding each component of the phrase, the enigma unravels, and what remains is a powerful concept that unites abstract theory with concrete utility. In the ensuing sections, we will explore how to implement and make practical use of Monads in Golang, a language not inherently functional but remarkably flexible. For a comprehensive implementation, feel free to check my monad package at GitHub.
The Basics of Monads
There are three fundamental laws a monad must obey:
- Left Identity:
return a >>= f
is the same asf(a)
- Right Identity:
m >>= return
is the same asm
- Associativity:
(m >>= f) >>= g
is the same asm >>= (\\x -> f x >>= g)
Here >>=
is the “bind” or “flat map” operator and return
is a function that puts a value into a monadic context.
Monad in Golang
Golang is not inherently functional, but you can borrow functional paradigms, especially since the introduction of generics at version 1.18. Here’s a simple example to simulate a monad:
type Maybe[T any] interface {
Nothing() bool
Value() T
FlatMap(func(T) Maybe[T]) Maybe[T]
}
type just[T any] struct {
val T
}
func Some[T any](x T) Maybe[T] {
return just[T]{val: x}
}
func (j just[T]) Nothing() bool {
return false
}
func (j just[T]) Value() T {
return j.val
}
func (j just[T]) FlatMap(f func(T) Maybe[T]) Maybe[T] {
return f(j.val)
}
type nothing[T any] struct{}
func None[T any]() Maybe[T] {
return nothing[T]{}
}
func (n nothing[T]) Nothing() bool {
return true
}
func (n nothing[T]) Value() T {
return *new(T)
}
func (n nothing[T]) FlatMap(_ func(T) Maybe[T]) Maybe[T] {
return n
}
The Maybe Monad
This is a simplified implementation of the Maybe Monad, which is often used to handle null or error states without breaking the function chain.
Example:
func half(n int) Maybe[int] {
if n%2 == 0 {
return Just(n/2)
}
return None[int]()
}
func main() {
result := Just(8).FlatMap(half).FlatMap(half)
if result.Nothing() {
fmt.Println("Invalid operation")
return
}
fmt.Println("Result:", result.Value()
}
Using Monads for Error Handling
Monads can also be applied in error handling. You can extend MaybeInt
to hold an error field and populate it when an error occurs.
type Result[T] interface {
Error() erro
Value() T
Failure() bool
FlatMap(func(T) Result[T]) Result[T]
}
type success[T] struct {
val T
}
func Succeed[T](val T) Result[T] {
return success[T]{val: val}
}
func (s success[T]) Error() error {
return nil
}
func (s success[T]) Value() T {
return s.val
}
func (s success[_]) Failure() bool {
return false
}
func (s success[T]) FlatMap(f func(T) Result[T]) Result[T] {
return f(s.val)
}
type failure[T] struct {
err error
}
func Fail[T](err error) Result[T] {
return failure[T]{err: err}
}
func (f failure[_]) Error() error {
return f.err
}
func (f failure[T]) Value() T {
return *new(T)
}
func (f failure[_]) Failure() bool {
return true
}
func (f failure[T]) FlatMap(_ func(T) Result[T]) Result[T] {
return f
}
Conclusion
While Golang is not designed to be a functional language, the monadic design pattern can still provide benefits. Adopting this style can lead to code that is easier to reason about, thereby reducing the chances of defects and increasing maintainability.
Remember, a Monad is not just a fancy concept; it’s a pattern that can make your code more efficient, cleaner, and more maintainable. So, the next time you find yourself in a complex state or error management situation, consider employing the monadic pattern.
Call to Action: Explore a Robust Implementation of Monads in Golang
If the abstract nature of monads has you yearning for a concrete implementation, I invite you to visit my GitHub repository dedicated to this topic: denisdubochevalier/monad. There, you’ll find a full, detailed implementation of monads (Maybe, Result, but also far more of them) in Golang, replete with examples and documentation.
This resource serves as an excellent complement to the foundational understanding presented here, offering practical utilities that you can integrate directly into your Go projects. Don’t merely treat monads as an academic curiosity — experience their utility and elegance in practice.
Leave a Reply